Demo
This section will be dedicated for examples that are using (mostly) what's learned in the guide.
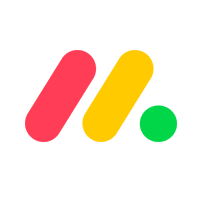
# Loader: Boxy
The Markup
styling
.loader {
width: 80px;
height: 80px;
position: relative;
}
.box {
position: absolute;
top: 0;
left: 0;
right: 50%;
bottom: 50%;
margin: 4px;
border: 12px solid #fff;
border-radius: 3px;
opacity: 0;
box-shadow: inset 2px 2px 2px 0px #00000057, 1px 1px 2px 0px #00000057;
}
.box_1 {
border-color: #ff844a;
z-index: 3;
animation: animateBoxLoaderPosition 4.5s ease-in-out infinite, animateBoxLoaderOpacity .25s ease-in-out forwards;
}
.box_2 {
border-color: #ffdf62;
z-index: 2;
animation: animateBoxLoaderPosition 4.5s ease-in-out 1.5s infinite, animateBoxLoaderOpacity 1s ease-in-out .25s forwards;
}
.box_3 {
border-color: #7de2af;
z-index: 1;
animation: animateBoxLoaderPosition 4.5s ease-in-out 3s infinite, animateBoxLoaderOpacity 1s ease-in-out 1.75s forwards;
}
Animation Rules
@keyframes animateBoxLoaderOpacity {
0% {
opacity: 0;
}
100% {
opacity: 1;
}
}
@keyframes animateBoxLoaderPosition {
0% {
top: 0;
left: 0;
right: 50%;
bottom: 50%;
}
6.25% {
top: 0;
left: 0;
right: 0;
bottom: 50%;
}
12.5%, 18.75%, 25% {
top: 0;
left: 50%;
right: 0;
bottom: 50%;
}
31.25% {
top: 0;
left: 50%;
right: 0;
bottom: 0;
}
37.5%, 43.75%, 50% {
top: 50%;
left: 50%;
right: 0;
bottom: 0;
}
56.25% {
top: 50%;
left: 0;
right: 0;
bottom: 0;
}
62.5%, 68.75%, 75% {
top: 50%;
left: 0;
right: 50%;
bottom: 0;
}
81.25% {
top: 0;
left: 0;
right: 50%;
bottom: 0;
}
87.5%, 93.75%, 100% {
top: 0;
left: 0;
right: 50%;
bottom: 50%;
}
}
# Effect: Liquid-Button
With hover effect.
The Markup
styling
.liquid_button {
position: relative;
padding: 20px 40px;
display: block;
text-decoration: none;
width: 200px;
overflow: hidden;
border-radius: 3px;
font-weight: 600;
}
.liquid_button > span {
position: relative;
z-index: 1;
color: #fff;
font-size: 20px;
letter-spacing: 8px;
}
.liquid_button > .liquid {
position: absolute;
left: 0px;
top: -80px;
width: 200px;
height: 200px;
background: #4973ff;
box-shadow: inset 0px 0px 50px rgba(0, 0, 0, .5);
transition: .5s;
}
.liquid_button:hover .liquid {
top: -120px;
}
.liquid_button > .liquid:before,
.liquid_button > .liquid:after {
content: '';
position: absolute;
width: 200%;
height: 200%;
top: 0;
left: 50%;
transform: translate(-50%, -75%);
}
.liquid_button > .liquid:before {
border-radius: 45%;
background: rgba(0, 0, 0, 1);
animation: liquidButton 5s linear infinite;
}
.liquid_button > .liquid:after {
border-radius: 40%;
background: rgba(0, 0, 0, .4);
animation: liquidButton 10s linear infinite;
}
Animation Rules
@keyframes animateLiquidButton {
0% {
transform: translate(-50%, -75%) rotate(0deg);
}
100% {
transform: translate(-50%, -75%) rotate(360deg);
}
}
# Effect: Image Clip-Path
With hover effect.
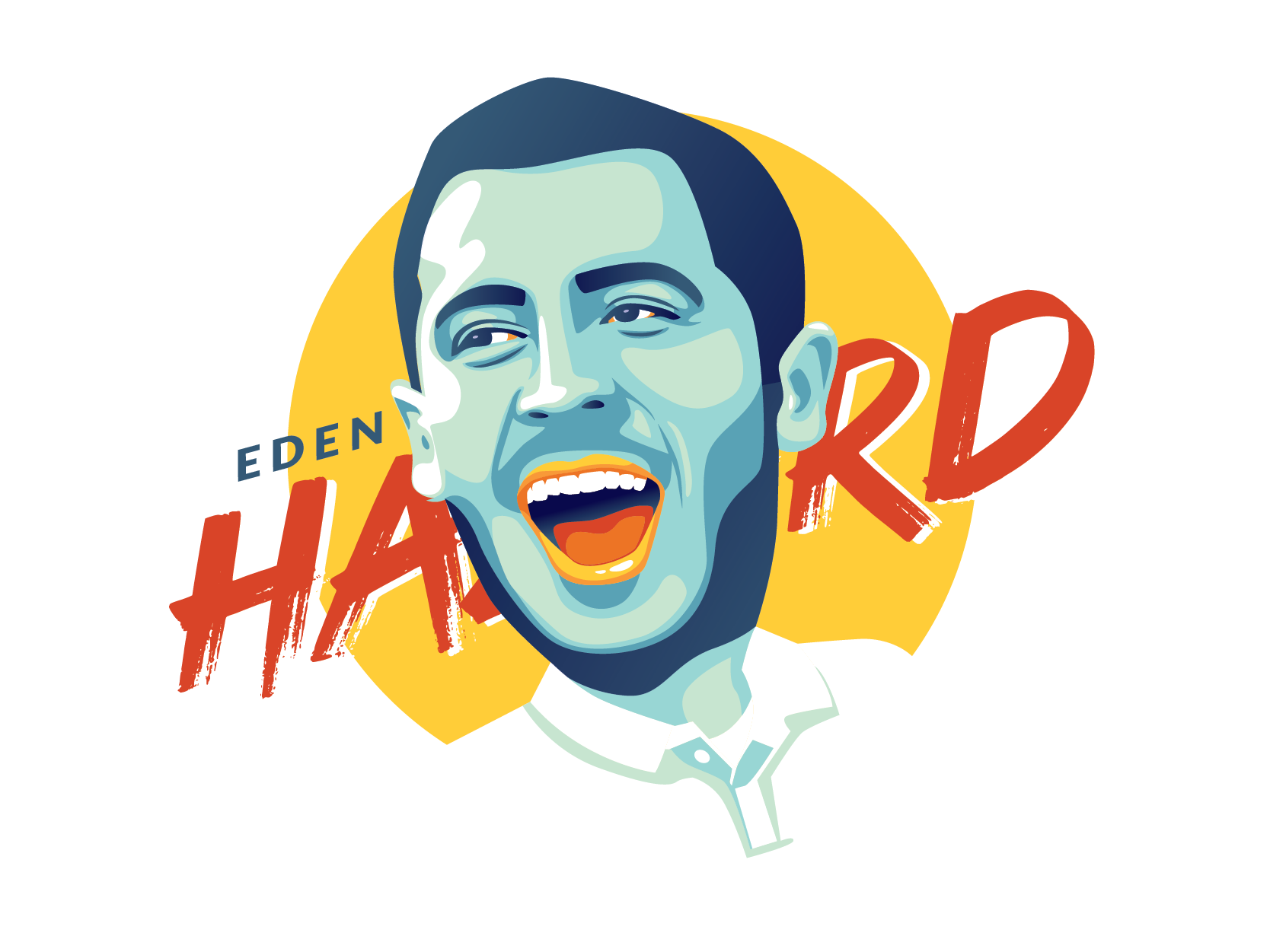
Lorem Ipsum is simply dummy text of the printing and typesetting industry, remaining essentially unchanged.
The Markup
styling
.card {
position: relative;
padding: 200px 24px 24px;
background: #fff;
box-sizing: border-box;
box-shadow: 0 1px 3px rgba(0, 0, 0, .1), 0 1px 2px rgba(0, 0, 0, .25);
overflow: hidden;
font-size: 14px;
border-radius: 3px;
width: 230px;
height: 350px;
}
.imageWrapper {
position: absolute;
top: 0;
bottom: 0;
left: 0;
right: 0;
transition: clip-path .4s;
overflow: hidden;
background: #fff;
clip-path: circle(30% at 50% 30%);
}
.imageWrapper:hover {
clip-path: circle(75% at 50% 50%);
}
.content {
height: 100%;
display: flex;
flex-direction: column;
justify-content: space-between;
text-align: center;
}
img {
height: 100%;
position: absolute;
left: 50%;
transform: translate(-50%, 0);
pointer-events: none;
user-select: none;
}
button {
width: 100%;
margin-top: 12px;
background: #491e5f;
box-shadow: 0px 0px 0px 0px #000;
font-weight: 600;
border: none;
padding: 4px 12px;
color: #fff;
border-radius: 3px;
font-family: inherit;
}
# Effect: Shining Text
Shining Text Animation Effects
The Markup
styling
.shining_text {
position: relative;
font-family: sans-serif;
text-transform: uppercase;
font-size: 2em;
letter-spacing: 4px;
overflow: hidden;
background: linear-gradient(90deg, #000, #fff, #000);
background-repeat: no-repeat;
background-size: 80%;
animation: animateShiningText 3s linear infinite;
-webkit-background-clip: text;
-webkit-text-fill-color: rgba(255, 255, 255, .01);
}
Animation Rules
@keyframes animateShiningText {
0% {
background-position: -500%;
}
100% {
background-position: 500%;
}
}