Grid
CSS Grid Layout is the most powerful layout system available in CSS. It is a 2-dimensional system, meaning it can handle both columns and rows, unlike flexbox which is largely a 1-dimensional system.
You work with Grid Layout by applying CSS rules both to a parent element (which becomes the Grid Container) and to that element's children (which become Grid Items).
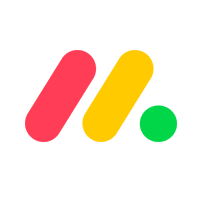
Grid container properties
# Display: Grid / Inline-Grid
This defines a grid container, inline or block depending on the given value. It enables a grid context for all of its direct children.
display: inline-grid does not make grid items display inline. It makes the grid container display inline.
# Grid-Template-Columns / Grid-Template-Rows
Defines the columns and rows of the grid with a space-separated list of values. The values represent the track size, and the space between them represents the grid line.
Naming rows and columns:
You can name rows and columns like in the example above:
[first-col] 25% [second-col] 150px [third-col] auto [fourth-col] 180px
This is optional, you can assign values only:
25% 150px auto 180px
the results will be the same, but in this case by default the rows and columns will be indexed and named by numbers which will be be equivalent to:
[1] 25% [2] 150px [3] auto [4] 180px.
It is recommended to give names for the row and columns because it will be easier and more declarative once you'll start positioning items in the grid (you can find examples in the grid-items properties).
Values can be defined in the following units: px / % / auto / fr (fraction - will be calculated as a portion of the available space).
The minmax(min, max) function:
Using this function, you can define a minimum and maximum for the specific column / row.
The repeat(numberOfTimes, length) function:
Using this function, you can define columns / rows that share the same length in a repeat method.
# Grid-Template-Areas
Defines a grid template by referencing the names of the grid areas which are specified with the grid-area property. Repeating the name of a grid area causes the content to span those cells. A period signifies an empty cell. The syntax itself provides a visualization of the structure of the grid.
# Grid-Template: (shortend property)
A shorthand property for setting the following properties in a single declaration:
grid-template-areas grid-template-rows / grid-template-columns.
# Grid-Row-Gap / Grid-Column-Gap
This properties creates gutters between columns and rows. Grid gaps are only created in between columns and rows, and not along the edge of the grid container.
# Grid-Gap: (shortend property)
A shorthand property for setting the following properties in a single declaration: grid-row-gap | grid-column-gap.
# Justify-Items
Aligns grid items along the inline (row) axis (as opposed to align-items which aligns along the block (column) axis). This value applies to all grid items inside the container.
# Align-Items
Aligns grid items along the block (column) axis (as opposed to justify-items which aligns along the inline (row) axis). This value applies to all grid items inside the container.
# Place-Items: (shortend property)
A shorthand property for setting the following properties in a single declaration: align-items | justify-items.
# Justify-Content
Sometimes the total size of your grid might be less than the size of its grid container. This could happen if all of your grid items are sized with non-flexible units like px. In this case you can set the alignment of the grid within the grid container. This property aligns the grid along the inline (row) axis.
# Align-Content
This property set the alignment of the grid rows within the grid container on the column axis if the grid items doesn't fill all the height of the grid container.
# Place-Content: (shortend property)
A shorthand property for setting the following properties in a single declaration: align-content | justify-content.
# Grid-Auto-Columns / Grid-Auto-Rows
This properties refers to auto created columns and rows when items were placed off the grid. this will let you set the size of those auto created columns and rows.
In this example, we have a 2X2 grid which creates 2 columns and 2 rows. Item 5 was placed in the second row (which exists) but in the fourth column (which does not exists), this creates two more columns, the columns that are created but not containing grid items (like column 3), will get 0px width, but the forth column that holds a grid item (item 5) will get an auto width size, which will be defined by its content.
Using the same example, here we are defining a grid-auto-columns of 105px which will set the third and the fourth auto created columns to that size.
Note: more about placing grid items in the grid items properties section.
# Grid-Auto-Flow
If you have grid items that you don't explicitly place on the grid, the auto-placement algorithm kicks in to automatically place the items. This property controls how the auto-placement algorithm works.
In the example above, items 1 and 6 are placed (explicitly) on the grid, and items 2 - 5 are placed in a row flow which is the default value.
Using the same example, here items 2 - 5 are placed on a column flow.
# Grid: (shortend property)
A shorthand for setting all of the following properties in a single declaration: grid-template-rows, grid-template-columns, grid-template-areas, grid-auto-rows, grid-auto-columns, and grid-auto-flow.
Grid items properties
# Grid-Column-Start / Grid-Column-End / Grid-Row-Start / Grid-Row-End
This properties defines the placement of the grid items on the grid itself using start and end points of the columns and rows. if you assigned names for your columns and rows you can refer to the start and end points by those names, otherwise you'll have to refer each row and column by their indexes (as numbers).
# Grid-column (shortend property)
A shorthand property for setting the following properties in a single declaration: grid-column-start / grid-column-end.
# Grid-Row: (shortend property)
A shorthand property for setting the following properties in a single declaration: grid-row-start / grid-row-end.
# Grid-Area
Gives an item a name so that it can be referenced by a template created with the grid-template-areas property.
Note: this property can also be used as a shorthand property for setting the following properties in a single declaration: grid-col-start / grid-col-end / grid-row-start / grid-row-end.
# Justify-Self
Aligns a grid item inside a cell along the inline (row) axis.
# Align-Self
Aligns a grid item inside a cell along the block (column) axis.
# Place-Self: (shortend property)
A shorthand property for setting the following properties in a single declaration: align-self / justify-self.